How to use the widget
How to place it on the page
A widget can be placed on a page in two ways.
An easy way
Just paste the following code into the page:
<script charset="utf-8" src="https://floors-widget.api.2gis.ru/loader.js" id="dg-floors-widget-loader"></script><script charset="utf-8">
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143'
}
});
</script>
The widget will appear on the page in the place where you inserted the code. This method is useful for simple static pages.
An advanced way
Include the widget JavaScript file in the head section of your page:
<script charset="utf-8" src="https://floors-widget.api.2gis.ru/loader.js" id="dg-floors-widget-loader"></script>
Add a div element to the page where you want to place the widget:
<div id="mywidget"></div>
Initialize the widget:
DG.FloorsWidget.init({
container: 'mywidget',
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143'
}
});
Notice the `container` — parameter — it accepts either the DOM element id or the DOM element itself. This method is useful for more complex cases where you need to create a dynamic widget after opening the page (by clicking a button, etc.).
Widget parameters
init method parameters
Parameter | Required | Description |
---|---|---|
width
|
yes | Widget width. Can be specified in pixels or percentage |
height
|
yes | Widget height. Can be specified in pixels or percentage |
container
|
no |
The element within which the widget should be created. You can pass either the element itself or its id. If not specified, the widget will be created where the initialization code is located (using document.write ).
|
initData
|
yes | Data for widget initialization |
Data for widget initialization (initData)
Parameter | Required | Description |
---|---|---|
complexId
|
yes | id of the building to show |
options
|
no | Widget options |
Widget options
Parameter | Description | Default value |
---|---|---|
locale
|
Locale of the widget interface. Available values: ru_RU , es_CL or en_US
|
ru_RU
|
initialSearchQuery
|
Initializes the widget containing search results for the specified query |
undefined
|
initialRubric
|
Initializes the widget containing the list of companies in the category with the specified id |
undefined
|
initialFirm
|
Initializes the widget containing the card of a company with the specified id |
undefined
|
initialZoom
|
Determines the zoom level at which the widget should be opened. If not specified, the scale is automatically selected to fit the entire building in the widget. This parameter is ignored if one of the parameters initialSearchQuery or initialRubric |
undefined
|
initialRotation
|
Specifies the rotation angle of the map at which the widget should be opened. Is specified in radians. If not specified, the angle is selected automatically |
undefined
|
initialFloor
|
Determines the floor at which the widget should be opened. If not specified, the default floor will be selected |
undefined
|
rotatable
|
Determines whether it is possible to rotate the map |
true
|
minZoom
|
Determines the minimum zoom level of the map. If not specified, it is calculated automatically |
undefined
|
maxZoom
|
Determines the maximum zoom level of the map. If not specified, it is calculated automatically |
undefined
|
Examples
// Specifies widget size in percent
DG.FloorsWidget.init({
width: '100%',
height: '100%',
initData: {
complexId: '141373143573143'
}
});
// Opens widget containing search results for the "clothes" query
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
initialSearchQuery: 'одежда'
}
}
});
// Opens the widget containing an open card of a cafe
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
initialFirm: '141265770712989'
}
}
});
// Opens the widget containing the "Coffee shops" category
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
initialRubric: '162'
}
}
});
// Opens the widget containing the Novosibirsk IKEA zoomed to 18
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143569821',
options: {
initialFirm: '141265769608851',
initialZoom: 18
}
}
});
// Opens the widget on floor -1
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
initialFloor: '-1'
}
}
});
// Prohibition to rotate the map
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
rotatable: false
}
}
});
// Custom scale boundaries
DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143',
options: {
minZoom: 16,
maxZoom: 25
}
}
});
Widget methods
DG.FloorsWidget.init
method returns an object with several methods available for calling.
Method | Description |
---|---|
search(query)
|
Opens the search results for the given query |
showFirm(firmId)
|
Opens the company card with the given id |
showRubric(rubricId)
|
Opens the list of companies in the category with the given id |
zoomIn()
|
Increases the zoom of the map |
zoomOut()
|
Decreases the zoom of the map |
showFloor(floor)
|
Switches the map to the given floor. Accepts a string with the floor name. |
Examples
var widget = DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143'
}
});
// Open the search results for "food" query
widget.search('Еда');
// Open the card of a firm
widget.showFirm('141265770712989');
// Open the "Coffee shops" category
widget.showRubric('162');
// Increase the zoom
widget.zoomIn();
// Switch floor to -2
widget.showFloor('-2');
Events
The widget object generates events that can be subscribed to. This allows you to execute some function when something happens with the widget. The following methods are used to manage events:
Method | Description |
---|---|
on(type, handler)
|
Adds a handler function to a particular event type
|
off(type, handler)
|
Removes a previously added handler from an event type
|
off(type)
|
Removes all handlers from an event type
|
off()
|
Removes all handlers from all event types |
The following event types are available:
Name | Description | Event data |
---|---|---|
init
|
Fired when the map is inited |
floorList , firmList
|
click
|
Fired when the user clicks a firm (a room or an icon) on the map |
firmIds
|
zoomend
|
Fired at the end of a zoom animation |
state
|
floorswitch
|
Fired when the displayed floor of the map changes |
state
|
Event data
floorList
Array containing all floor names in the bottom-to-top order
firmList
Array containing all firms in the building. Elements of this array are objects with the following properties:
Name | Type | Description |
---|---|---|
id
|
string
|
Firm id |
floor
|
string
|
Name of the floor on which the firm is located |
state
Object containing data about the current state of the map. Has the following properties:
Name | Type | Description |
---|---|---|
floor
|
string
|
Name of the current floor |
zoom
|
number
|
Current zoom |
minZoom
|
number
|
Lower zoom limit |
maxZoom
|
number
|
Upper zoom limit |
firmIds
Array containing ids of the firms that have been clicked. Can't be empty. Can have more than one element if the user clicked a room with multiple firms in it.
Examples
const widget = DG.FloorsWidget.init({
width: '960px',
height: '600px',
initData: {
complexId: '141373143573143'
}
});
// 'init' event
widget.on('init', (event) => {
console.log(event.type); // 'init'
console.log(event.floorList); // ['-2', '-1', '1', '2', '3', '4']
console.log(event.firmList); // [{id: '12345', floor: 0, floorName: '-2'}, ...]
});
// 'click' event
widget.on('click', (event) => {
console.log(event.type); // 'click'
console.log(event.firmIds); // ['1234', '5678']
});
// 'zoomend' event
widget.on('zoomend', (event) => {
console.log(event.type); // 'zoomend'
console.log(event.state); // {floor: 2, zoom: 18, minZoom: 17.5, maxZoom: 21.6}
});
// 'floorswitch' event
widget.on('floorswitch', (event) => {
console.log(event.type); // 'floorswitch'
console.log(event.state); // {floor: 3, zoom: 18, minZoom: 17.5, maxZoom: 21.6}
});
// Adding an event handler and removing it
const handler = (event) => console.log(event);
widget.on('click', handler);
widget.off('click', handler);
// Removing all handlers from 'click' event
widget.off('click');
// Removing all handlers from all events
widget.off();
How to find object ids
Building id
-
1. Open the card of a building you need on 2gis.ru
-
2. Hover your mouse over the address
-
3. id will be displayed in the tooltip that appears
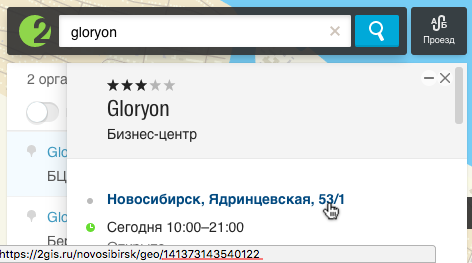
Company id
-
1. Open the card of a company you need on 2gis.ru
-
2. id will be displayed in the address bar
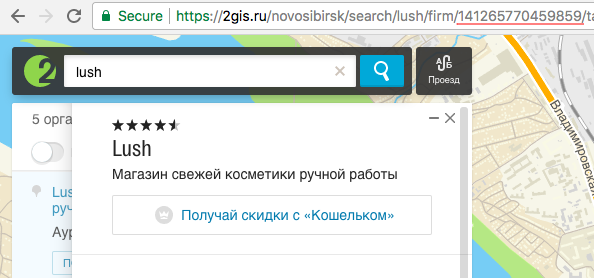
Category id
-
1. Open 2gis.ru
-
2. Open "All categories"
-
3. Select the category you need and open it
-
4. id will be displayed in the address bar
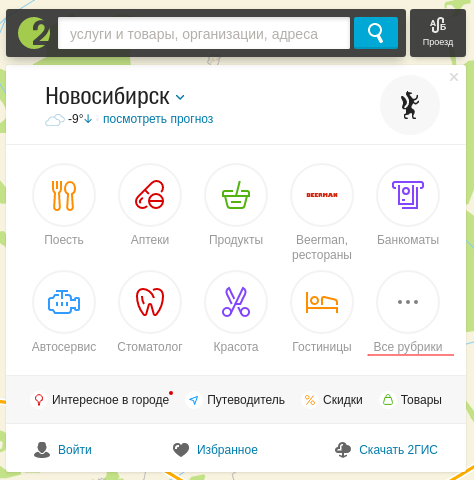
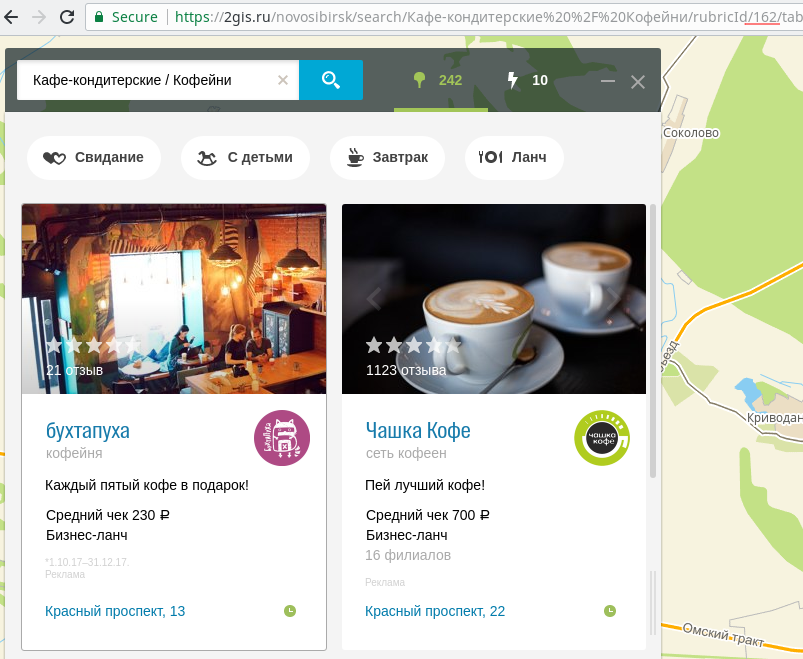